Table of Contents
Introduction
The Code-First approach in Entity Framework allows developers to define database schemas directly through C# or VB.NET classes. This method empowers developers to focus on domain modeling without worrying about the underlying database design, making it a preferred choice in agile development environments.
In this blog, we will explore the Code-First approach in Entity Framework, its features, implementation, and how it compares to other approaches like Database-First and Model-First. By the end, you’ll have a clear understanding of how to create and manage databases efficiently using Code-First principles.
The following diagram illustrates Code-First Approach in Entity Framework guide:
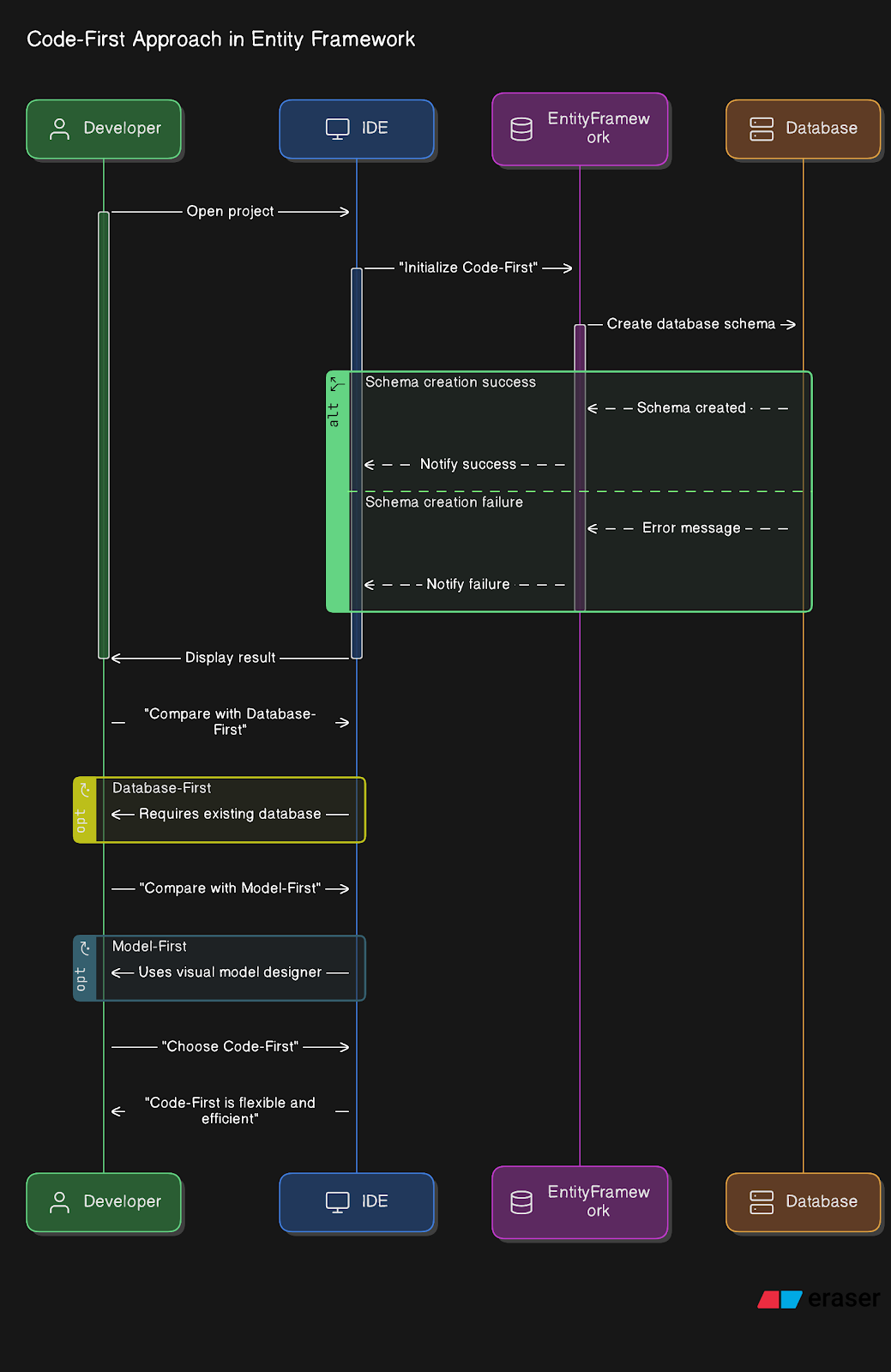
What is the Code-First Approach?
The Code-First approach in Entity Framework enables developers to define the structure of their database directly through classes and properties in the application code. Entity Framework generates the database schema based on these classes, removing the need for manual database design.
This approach is particularly useful for developers who prefer to work with code rather than database design tools, allowing for better integration with source control and easier updates to the schema over time.
Key Features of the Code-First Approach
The Code-First approach offers several powerful features that make it a favorite among developers:
- Model-Driven Development: Define database structures through C# or VB.NET classes.
- Flexible Configuration: Use annotations or Fluent API for custom configurations.
- Migrations: Seamlessly manage database schema changes without data loss.
- Dependency Injection: Integrate with dependency injection frameworks for better testability.
- Cross-Platform Support: Works seamlessly with .NET Core for cross-platform applications.
Advantages of Code-First Development
The Code-First approach offers several advantages over other methods:
- Developer-Centric: Focuses on writing code rather than designing databases, speeding up development.
- Version Control Friendly: Schema changes are managed through migrations, which can be tracked in source control.
- Flexibility: Enables quick schema updates without manual intervention.
- Integration: Works seamlessly with dependency injection, unit testing, and other modern practices.
Step-by-Step Guide to Code-First in Entity Framework
Let’s walk through the process of implementing the Code-First approach in an ASP.NET Core application.
- Create a New Project: Use Visual Studio or the .NET CLI to create an ASP.NET Core Web Application.
-
Install Entity Framework Core:
Install EF Core packages using NuGet:
dotnet add package Microsoft.EntityFrameworkCore dotnet add package Microsoft.EntityFrameworkCore.SqlServer dotnet add package Microsoft.EntityFrameworkCore.Tools
-
Define Your Models:
Create C# classes to represent your database tables:
public class Product { public int ProductId { get; set; } public string Name { get; set; } public decimal Price { get; set; } }
-
Create the DbContext:
Define a DbContext class to manage the connection to the database:
public class ApplicationDbContext : DbContext { public DbSet<Product> Products { get; set; } protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) { optionsBuilder.UseSqlServer("YourConnectionString"); } }
-
Apply Migrations:
Use the CLI to create and apply migrations:
dotnet ef migrations add InitialCreate dotnet ef database update
Using Migrations in Code-First
Migrations allow you to manage changes to your database schema over time. They generate code to apply incremental updates, making it easy to evolve your database alongside your application.
Here’s how to manage migrations:
- Add a Migration: Use
dotnet ef migrations add
to create a new migration. - Apply Changes: Run
dotnet ef database update
to apply changes to the database. - Roll Back Changes: Use
dotnet ef database update PreviousMigration
to roll back to a specific migration.
Best Practices for Code-First Approach
- Use Descriptive Names: Name your classes and properties meaningfully to reflect the database structure.
- Regular Backups: Backup your database before applying migrations to prevent data loss.
- Use Fluent API: For complex configurations, use the Fluent API for better readability and control.
- Automated Testing: Integrate automated tests to verify schema changes.
Common Issues and Troubleshooting
While using the Code-First approach, you might encounter issues like migration conflicts, data loss, or performance bottlenecks. Here are some solutions:
- Migration Conflicts: Resolve conflicts by merging migration files manually.
- Data Loss: Always backup data before applying migrations.
- Slow Performance: Optimize queries and use lazy loading judiciously.
Conclusion
The Code-First approach in Entity Framework is a powerful and flexible way to manage database development in .NET applications. It simplifies the process of defining and evolving schemas, integrates seamlessly with modern development workflows, and improves productivity.
Start leveraging the Code-First approach today to build scalable, maintainable, and robust .NET applications.